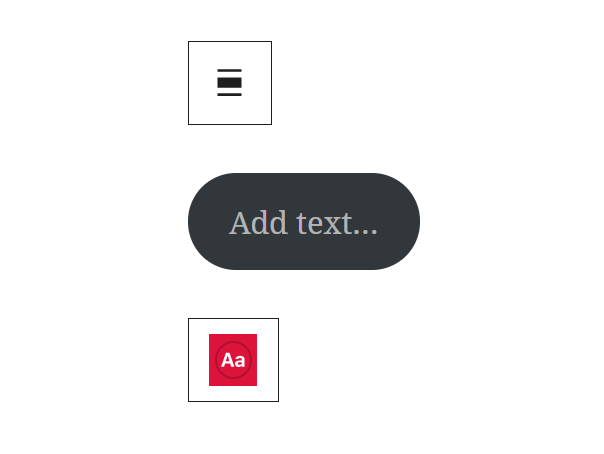
Can be used to replicate another block inside a block. The block can then be changed to fit the current context.
import { registerBlockType } from '@wordpress/blocks';
import { __ } from '@wordpress/i18n';
import {
useBlockProps,
BlockEdit,
} from '@wordpress/block-editor';
//styles that make it look good in the editor
import './editor.scss';
const BLOCKNAME = "block-edit";
const BLOCKPATH = `wp-gb/${BLOCKNAME}`;
registerBlockType( BLOCKPATH, {
apiVersion: 2,
title: __( BLOCKNAME.replace("-", " ").toUpperCase(), 'wp-gb' ),
description: __( 'The description' ),
category: 'wp-gb',
icon: 'smiley',
attributes: {
align: {
type: "string",
},
content: {
type: "string",
},
},
edit: ( {attributes, setAttributes, clientId, isSelected} ) => {
return (
<div { ...useBlockProps() }>
<BlockEdit
name="wp-gb/block-alignment-control"
attributes={ { align: attributes.align } }
setAttributes={ setAttributes }
clientId={clientId}
isSelected={isSelected}
/>
<BlockEdit
name="core/button"
attributes={ { content: attributes.content } }
setAttributes={ setAttributes }
clientId={clientId}
isSelected={isSelected}
/>
<BlockEdit
name="wp-gb/block-colors-style-selector"
attributes={ {} }
clientId={clientId}
isSelected={isSelected}
/>
</div>
)
},
} );
isSelected and clientId is needed in order to make keep focus on a BlockEdit block if there i multiple of them.
Attributes that is updated needs to have the exact same name inside the block itself. So if the attribute that is updated in wp-gb/block-alignment-control is “align”, then the attribute that is sent with the attributes prop needs to have the name “align”.
setAttributes is added as a prop because the replecated block needs to know which setAttributes function it should use when updating attributes.
The attribute and name prop is required.