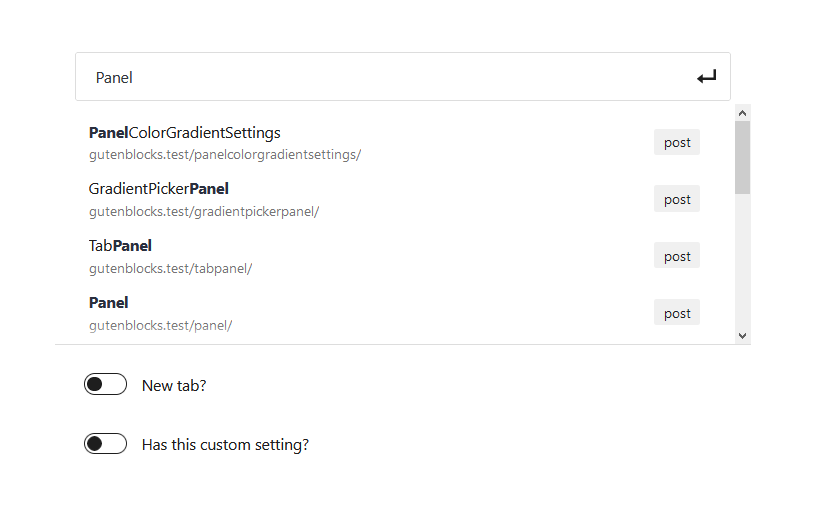
Used to search for any url on the site. External url can also be created. Used when creating link in a core paragraph block by WordPress for example.
import { registerBlockType } from '@wordpress/blocks';
import { __ } from '@wordpress/i18n';
import {
useBlockProps,
__experimentalLinkControl as LinkControl
} from '@wordpress/block-editor';
//styles that make it look good in the editor
import './editor.scss';
const BLOCKNAME = "link-control";
const BLOCKPATH = `wp-gb/${BLOCKNAME}`;
registerBlockType( BLOCKPATH, {
apiVersion: 2,
title: __( BLOCKNAME.replace("-", " ").toUpperCase(), 'wp-gb' ),
description: __( 'The description' ),
category: 'wp-gb',
icon: 'smiley',
attributes: {
post: {
type: "object",
}
},
edit: ( {attributes, setAttributes} ) => {
return (
<div { ...useBlockProps() }>
<LinkControl
searchInputPlaceholder="Search here..."
value={ attributes.post }
settings={[
{
id: 'opensInNewTab',
title: 'New tab?',
},
{
id: 'customDifferentSetting',
title: 'Has this custom setting?'
}
]}
onChange={ ( newPost ) => setAttributes( { post: newPost } ) }
withCreateSuggestion={true}
createSuggestion={ (inputValue) => setAttributes( { post: {
...attributes.post,
title: inputValue,
type: "custom-url",
id: Date.now(),
url: inputValue
} } ) }
createSuggestionButtonText={ (newValue) => `${__("New:")} ${newValue}` }
>
</LinkControl>
</div>
)
},
} );
You can also get the __experimentalLinkControlSearchInput, __experimentalLinkControlSearchResults and __experimentalLinkControlSearchItem components if you want to dig deep into this module. However you would have to understand how they are used in the GitHub repository by Gutenberg. You can for example use the __experimentalLinkControlSearchInput to render your custom suggenstions drop down like this:
import { registerBlockType } from '@wordpress/blocks';
import { __ } from '@wordpress/i18n';
import {
useBlockProps,
__experimentalLinkControlSearchInput as LinkControlSearchInput
} from '@wordpress/block-editor';
//styles that make it look good in the editor
import './editor.scss';
const BLOCKNAME = "link-control-search-input";
const BLOCKPATH = `wp-gb/${BLOCKNAME}`;
registerBlockType( BLOCKPATH, {
apiVersion: 2,
title: __( BLOCKNAME.replace("-", " ").toUpperCase(), 'wp-gb' ),
description: __( 'The description' ),
category: 'wp-gb',
icon: 'smiley',
attributes: {
url: {
type: "string",
}
},
edit: ( {attributes, setAttributes} ) => {
const suggestionsRender = (props) => (
<div className="components-dropdown-menu__menu">
{ props.suggestions.map( ( suggestion, index ) => {
return (
<div onClick={ () => props.handleSuggestionClick( suggestion ) } className="components-button components-dropdown-menu__menu-item is-active has-text has-icon">{suggestion.title}</div>
)
} )
}
</div>
)
return (
<div { ...useBlockProps() }>
<LinkControlSearchInput
placeholder="Search here..."
renderSuggestions={ ( props ) => suggestionsRender(props) }
allowDirectEntry={false}
withURLSuggestion={false}
value={ attributes.url }
onChange={ ( newURL ) => setAttributes( { url: newURL } ) }
withCreateSuggestion={false}
/>
</div>
)
},
} );